We
Open Source
A community education resource
Celebrating 60 years of BASIC and why it’s still relevant for new programmers
BASIC was the starting point to learn about computer programming.
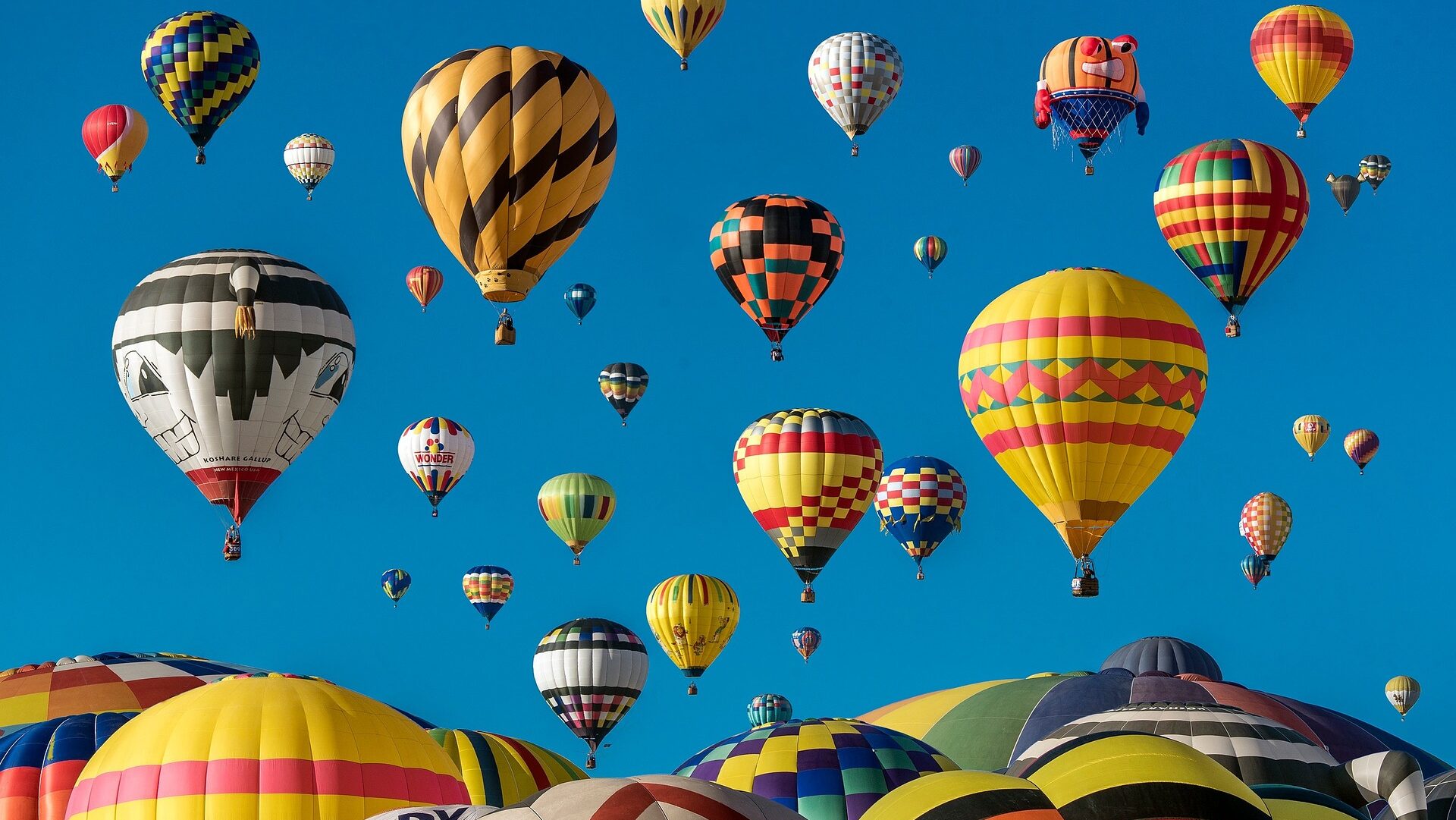
The BASIC programming language turned sixty years old this month. As Dartmouth College shares in a history of BASIC, the first demonstration of BASIC was May 1, 1964. BASIC made it easy for anyone to get started with computers by writing their first program.
BASIC, the Beginner’s All-purpose Symbolic Instruction Code, lets you make the computer do something new (at least, to you). One reason BASIC was so easy to use was it was interpreted rather than compiled, so you could easily switch between writing your program and running it. If the program aborted, you could see right where it stopped and directly edit the code to fix it, then run the program from where it left off.
BASIC became a popular programming language, especially for beginners. So it is not a surprise that early personal computers came bundled with their own version of BASIC. The TRS-80, Commodore PET, and Apple II computers came out in 1977, when I was just five years old. These computers were simple by today’s standards, with memory measured in kilobytes, but they promised a golden era of a computer in every classroom and every home. My elementary school purchased a “lab” of two Apple II computers, and my parents bought an Apple II clone that my brother and I learned how to use.
All of these computers came with BASIC. I thought this was incredible. My brother and I taught ourselves how to use BASIC to write more interesting and more sophisticated programs. I started with simple math quizzes before graduating to more advanced programs like puzzle games and adventure games.
Table of contents
- Getting started with GW-BASIC
- Our first BASIC program
- A simple loop
- A guessing game
- Great for new programmers
Getting started with GW-BASIC
When my family eventually replaced the Apple II at home, we bought an IBM Personal Computer. And you guessed it – the IBM PC also came with BASIC. The first version of IBM BASIC was called BASICA, and required a special hardware cassette. A later version called GW-BASIC was identical to BASICA but ran entirely as software.
I liked programming in GW-BASIC when I was growing up. BASIC was my “gateway” to computer programming, and will always be special to me. So I was excited in 2020 when Microsoft released GW-BASIC as open source software under the MIT license. You can find the source code at GW-BASIC on GitHub.
It didn’t take long for others to dig into the source code and get it working on modern systems. One excellent example is T.K. Chia, et al’s GW-BASIC who first got it working on FreeDOS. You can download the latest release from 2022 and run it to see what it was like to use BASIC in these early days.
Our first BASIC program
Unzip the GWB22C16.ZIP
package on FreeDOS, and run the GWBASIC
program. This brings up the BASIC interpreter:
GW-BASIC 2022-12-16 version (JWasm), MIT License
(C) Copyright D. Spinellis, S. Gros, T.K. Chia 2020--2022
(C) Copyright Microsoft 1982
62618 Bytes free
Ok
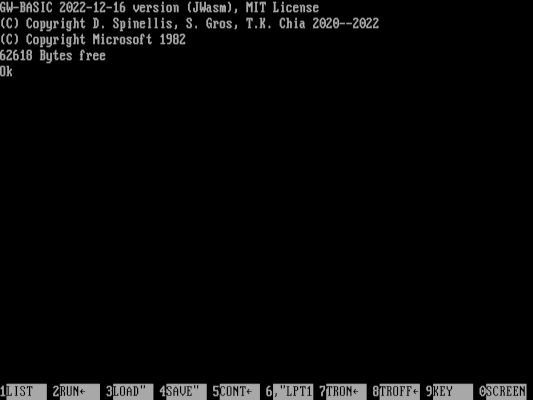
One simple program just prints the text “Hello world” and then exits. This is trivial to write:
10 PRINT "Hello world"
One thing to note is that BASIC uses line numbers for every instruction. As a young programmer, I learned to enter each instruction with line numbers like 10, 20, 30, 40, and so on. By “going up” by tens, I had room if I needed to add new instructions between two other lines, like a line 21, 22, 23, … up to line 29.
To run the program, just type the RUN
instruction and hit Enter:
run
Hello world
Ok
But this is just a quick program to demonstrate how to print text. If we don’t need it anymore, we can clear the program listing and start a new program with the NEW command:
new
Ok
A simple loop
Let’s say we wanted to print the numbers from one to ten. We can do that in a few ways. One way is to store 1 in a variable, then increment it every time we print it, and stop when we reach 10
. That program might look like this:
10 let i = 1
20 print i
30 let i = i + 1
40 if i < 11 then goto 20
50 end
list
10 LET I = 1
20 PRINT I
30 LET I = I + 1
40 IF I < 11 THEN GOTO 20
50 END
Ok
The first half of that listing is me typing in the program. BASIC is case-insensitive when you are entering program instructions, so I have typed this using lowercase letters. Afterwards, I used the LIST
instruction to have GW-BASIC print my program listing so I can make sure it is correct.
This program stores 1
into the variable I
on line 10, then prints the value on line 20. Line 30 adds one to the variable. On line 40, I test if the variable I
is less than eleven, and if so (only for values from one to ten) jumps back to line 20 to print the value and start over. When the variable I
reaches 11, the program continues to line 50, which ends the program. In all, the program prints the values from one to ten:
run
1
2
3
4
5
6
7
8
9
10
Ok
Another way to do this is with the FOR
loop. This is the most efficient way to loop a variable over a set of values. Let’s demonstrate by starting a new program that uses FOR
to print the values from one to ten:
new
Ok
10 for i = 1 to 10
20 print i
30 next i
list
10 FOR I = 1 TO 10
20 PRINT I
30 NEXT I
Ok
This is a short program! And the FOR
statement makes this much easier to read: it just prints the values from one to ten:
run
1
2
3
4
5
6
7
8
9
10
Ok
You can also “nest” multiple FOR
instructions to create more complex instructions. For example, to print all possible combinations of two lists of 1, 2, and 3, we can write a program like this:
Ok
10 for i = 1 to 3
20 for j = 1 to 3
30 print i,j
40 next j
50 next i
list
10 FOR I = 1 TO 3
20 FOR J = 1 TO 3
30 PRINT I,J
40 NEXT J
50 NEXT I
Ok
This program iterates over two lists of 1, 2, and 3 and prints every possible combination. With three numbers in each list, this is nine possible combinations:
run
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Ok
You can make this list slightly easier to read by changing line 30, to not use the comma:
30 print i j
list
10 FOR I = 1 TO 3
20 FOR J = 1 TO 3
30 PRINT I J
40 NEXT J
50 NEXT I
Ok
The comma effectively adds a tab between each value. Without the comma, GW-BASIC doesn’t print anything between them, although it appears like a space in this example because GW-BASIC prints these small numbers as space-padded:
run
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Ok
A guessing game
Let’s write something a little more complex to celebrate sixty years of BASIC. For this program, we can write a simple number-guessing game. The program will pick a random number between 1 and 100, and we need to guess it. At every guess, the program will tell us if our guess is too low or too high, until we get it right:
new
Ok
10 let s = int(rnd(1)*100)+1
20 print "Guess the secret number from 1 to 100:"
30 input g
40 if g < s then print "Too low"
50 if g > s then print "Too high"
60 if g <> s then goto 30
70 print "That's right!"
80 end
list
10 LET S = INT(RND(1)*100)+1
20 PRINT "Guess the secret number from 1 to 100:"
30 INPUT G
40 IF G < S THEN PRINT "Too low"
50 IF G > S THEN PRINT "Too high"
60 IF G <> S THEN GOTO 30
70 PRINT "That's right!"
80 END
Ok
This program introduces several new concepts about BASIC programming. The RND
function generates a random value between zero and one, but never quite gets to one. Technically, this is called a “floating point” value from 0.0 to 0.999… We can turn that into a value from 0 to 99 by multiplying the random number by 100. Mathematically, that gives a value from 0.0 to 99.999… We can turn that into an integer value with the INT
function, giving a value between 0 to 99. Adding 1 to this means the secret number in the S variable will be between 1 and 100.
Line 20 prints the rules of the game, and line 30 asks the user to enter a value, which gets saved in the G
(guess) variable. Lines 40 and 50 tell the user if their guess was too low or too high. Line 60 jumps back to line 30 (to input another guess) only if the guess is not equal to the secret number. Otherwise, the program congratulates the user before ending.
run
Guess the secret number from 1 to 100:
? 50
Too high
? 25
Too high
? 15
Too high
? 10
Too low
? 12
Too low
? 14
Too high
? 13
That's right!
Ok
Every time we run the program, however, the random number gets set to the same value:
run
Guess the secret number from 1 to 100:
? 13
That's right!
Ok
run
Guess the secret number from 1 to 100:
? 13
That's right!
Ok
To fix this, we need to use the RANDOMIZE
instruction before generating a random value with RND
:
1 randomize
list
1 RANDOMIZE
10 LET S = INT(RND(1)*100)+1
20 PRINT "Guess the secret number from 1 to 100:"
30 INPUT G
40 IF G < S THEN PRINT "Too low"
50 IF G > S THEN PRINT "Too high"
60 IF G <> S THEN GOTO 30
70 PRINT "That's right!"
80 END
Ok
And now every time we run the program, it prompts us for a new random number seed that GW-BASIC uses to select a completely new random value for the secret number. This is much more fun to play:
run
Random number seed (-32768 to 32767)? 3456
Guess the secret number from 1 to 100:
? 13
Too low
? 50
Too high
? 30
Too low
? 40
Too low
? 45
Too low
? 47
Too low
? 48
That's right!
Ok
When you’re done with your program, just use the SYSTEM
command to exit the GW-BASIC environment.
Great for new programmers
If you are new to programming, BASIC can be an easy first step. While using line numbers may seem primitive compared to modern programming languages, the tradeoff is that the interpreter makes it easy to experiment and pick up where you left off. And “retro” programming is fun, too. Give BASIC a try if you want to experiment with this classic way to create programs.
The opinions expressed on this website are those of each author, not of the author's employer or All Things Open/We Love Open Source.