We ❤️ Open Source
A community education resource
How to write your first FreeDOS program
Learn how to compile, run, and customize your FreeDOS code.
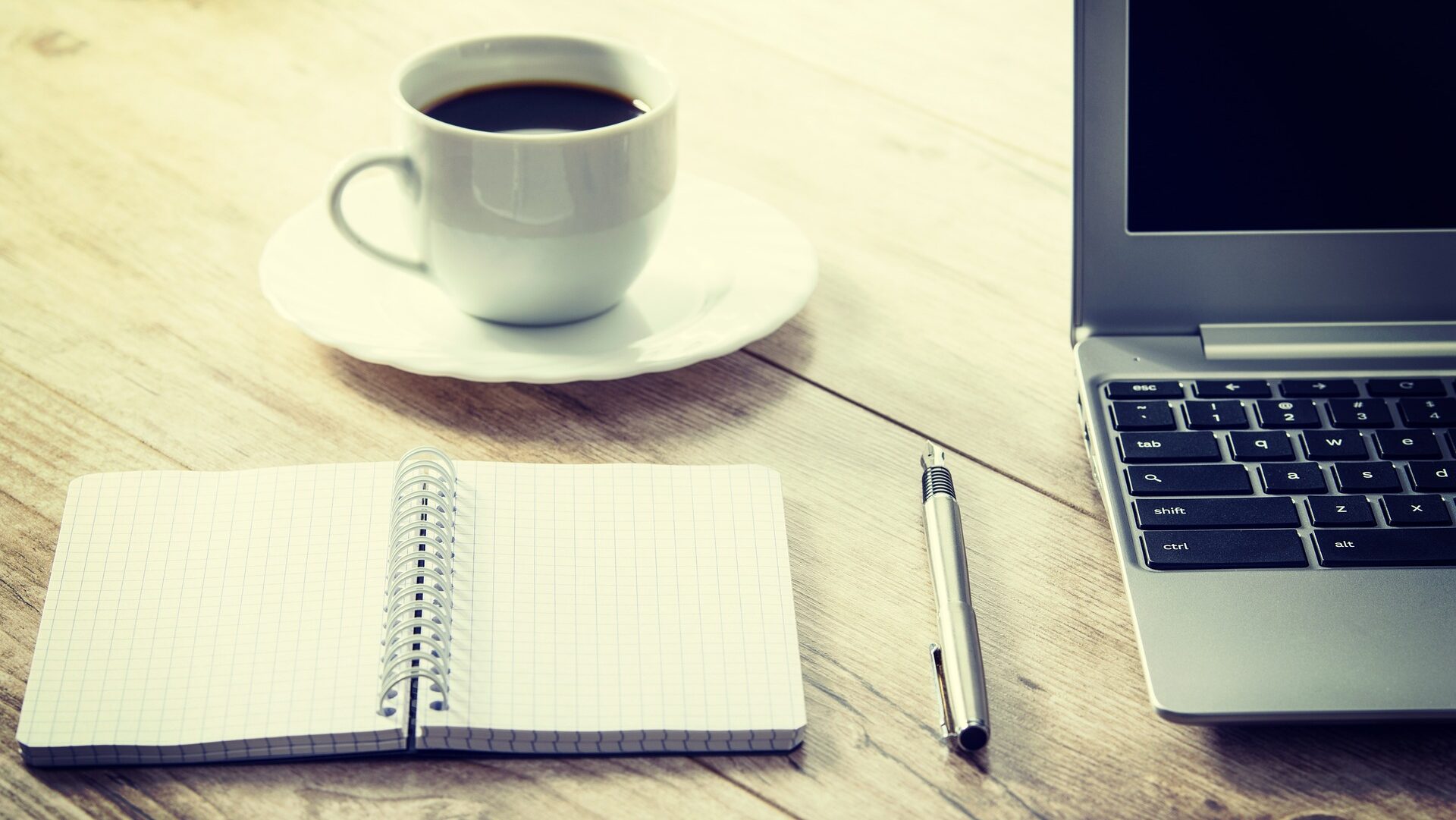
I like to have fun with programming. It’s why I got “into” computers at a young age; the ability to make your own program that meets your specific need and solves a problem you have is what makes programming exciting.
A bit of programming also makes “retrocomputing” a fun activity. If you know how to create your own programs, you can add more modern tools to these “classic” operating systems. Let’s explore how to write your first program on my favorite retrocomputing project: FreeDOS.
FreeDOS is a modern reimplementation of the classic DOS operating system. During its peak in the 1980s and 1990s, DOS didn’t have a very powerful command line. You had to create your programs to extend the features of the DOS command line to add new features. While FreeDOS includes many modern tools, it’s still nice to know how to make your own programs.
Pick your favorite compiler
FreeDOS provides a wide variety of compilers and assemblers that you can use to create your own programs. For example, C programmers might be interested in Open Watcom C, BCC (“Bruce’s C Compiler”), or the IA-16 version of the GNU C Compiler. These are all available on the “Bonus CD” in the FreeDOS distribution.
For this demonstration, let’s use the Open Watcom C compiler, which is our preferred C compiler for creating official FreeDOS programs. Make sure you have the “Bonus CD” in your CD drive, or connect the FD13BNS.iso file to your virtual machine, then run the FDIMPLES
program (“FreeDOS Installer – My Package List Editor Software”). Navigate to the “Development” package group and install the “Open Watcom C/C++ compiler” package.
Read more: 5 FreeDOS editors I love
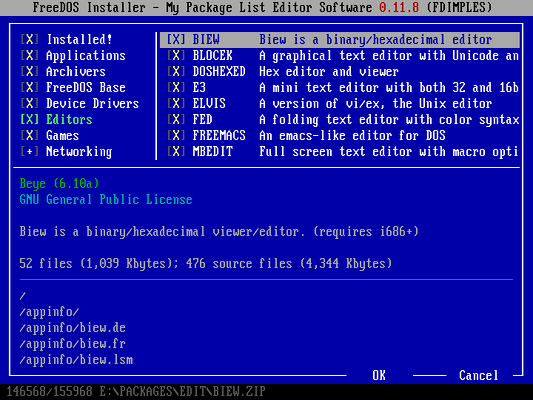
The Open Watcom C compiler is installed to the C:\DEVEL\WATCOMC
directory. But because DOS has limited memory, most developers would not add every installed program to the PATH
variable. Instead, it’s more typical to only add the path and set up other environment variables when you plan to use the compiler to make new programs.
You can use the OWSETUP.BAT
batch program to set up your environment to use the Open Watcom C compiler package. This sets the PATH
variable and a few other environment variables that Open Watcom needs to find its files. The batch file looks like this:
@echo off
if "%WATCOM%" == "" goto SetEnv
if not exist %DOSDIR%\BIN\VFDUTIL.COM goto SetEnv
VFDUTIL /T WFC.EXE /T WFL.EXE
if errorlevel 1 goto SetEnv
goto End
:SetEnv
echo Open Watcom C/C++ Build Environment
SET PATH=C:\DEVEL\WATCOMC\BINW;%PATH%
SET INCLUDE=C:\DEVEL\WATCOMC\H;%INCLUDE%
SET WATCOM=C:\DEVEL\WATCOMC
SET EDPATH=C:\DEVEL\WATCOMC\EDDAT
SET WIPFC=C:\DEVEL\WATCOMC\WIPFC
:End
Navigate to the C:\DEVEL\WATCOMC
directory and run the OWSETUP
command:
C:\DEVEL\WATCOMC>owsetenv.bat
Open Watcom C/C++ Build Environment
Pick your favorite editor
FreeDOS includes a variety of text editors that you can use to create source code for programs. Everyone has their own favorite editor, and I have a few favorites that I keep using all the time, such as Fed (the folding editor), Freemacs, or Open Watcom’s Vi. You can install all of these using the FDIMPLES
package manager, in the “Editors” package group.
Fed is my favorite editor for FreeDOS programming. Fed stands for Folding editor, because it can “fold” a block of code in a source file. This helps to remove distracting code that you don’t need to see right away, even large blocks such as functions. Fed lets you change all the colors in the editor. I prefer to edit my FreeDOS programs with white text on a blue background, with comments in bright blue so they almost “fade away” so they don’t get in my way.
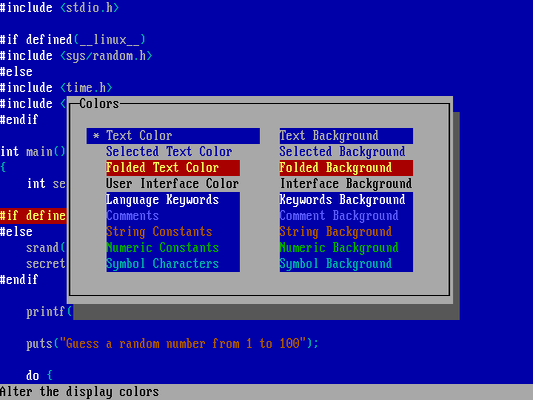
If you’re coming to FreeDOS as a Linux user, you might be more comfortable with Open Watcom’s Vi, which provides a classic-yet-updated implementation of the venerable Vi editor. You can configure Vi with a custom “startup” file to make it look and act more like classic Vi, or use the defaults to use the menus, tiled windows, and other updated features.
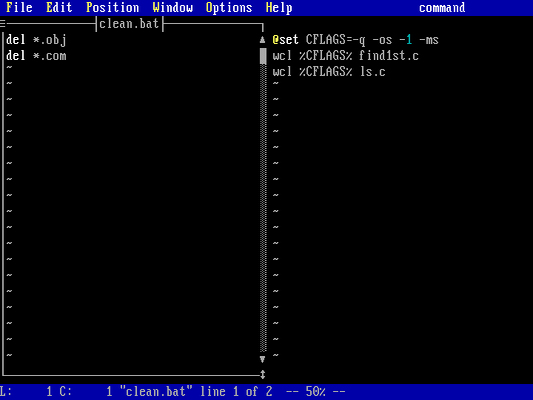
Counting numbers
With our favorite text editor, and with the environment set up to use the C compiler, we can now write our own program. Let’s write a simple program that counts numbers, similar to the seq
command on Linux, which generates a sequence of numbers. This is a useful tool to have on-hand if you need to generate predictable input to test other programs.
Our simplified seq
command accepts several values from the command line: a starting value, an ending value, and an increment to get there. We’ll use the atoi()
standard C library function to convert the command line values to integers.
This uses a switch
statement that “falls through” each condition. If the argument “count” (argc
) is 4 (the program name plus 3 options) then it saves the step
value .. then continues to the 3 condition (the program name plus 2 options) to get the stop
value .. and finally passes to the 2 condition (the program name plus 1 option) to save the start
value.
The program then does simple bounds checking to ensure the sequence can only count “up” before printing the list of numbers:
#include <stdio.h>
#include <stdlib.h> /* atoi */
int main(int argc, char **argv)
{
int start = 1, stop = 10, step = 1;
int i;
if (argc > 4) {
puts("too many options!");
puts("usage: seq [start [stop [step]]]");
return 1;
}
/* get values */
switch(argc) {
case 4: step = atoi(argv[3]);
case 3: stop = atoi(argv[2]);
case 2: start = atoi(argv[1]);
}
/* simple bounds checking */
if ((stop<start) || (step<1)) {
puts("cannot count 'down'");
return 2;
}
/* make the sequence */
for (i = start; i <= stop; i += step) {
printf("%d\n", i);
}
return 0;
}
Save this as seq.c
on your FreeDOS system.
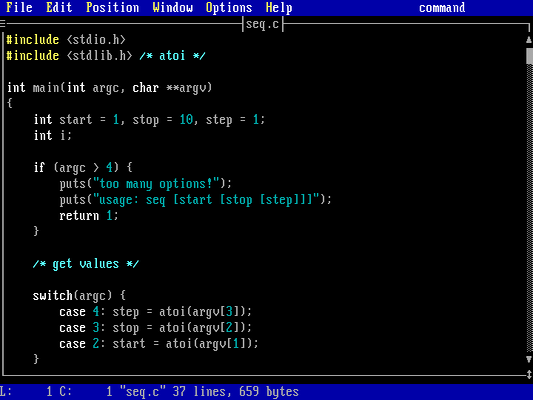
Compile and run the program
Now compile the program using the Open Watcom C compiler. Open Watcom tends to write a lot of output during the compilation process, so I usually add the -q
(quiet) option to keep it from printing anything that I don’t need. All that’s left is the output from the DOS “extender” program, which is just informational:
C:\DEVEL\WATCOMC>wcl -q seq.c
DOS/4GW Protected Mode Run-time Version 1.97
Copyright (c) Rational Systems, Inc. 1990-1994
DOS/4GW Protected Mode Run-time Version 1.97
Copyright (c) Rational Systems, Inc. 1990-1994
If you want to prevent the DOS4G extender from printing any output at all, set the DOS4G
environment variable to quiet
, and then the compilation process won’t print anything unless there’s an error or warning:
C:\DEVEL\WATCOMC>set DOS4G=quiet
C:\DEVEL\WATCOMC>wcl -q seq.c
Congratulations, you’ve written your first FreeDOS program! You can use your new seq
command to generate a list of numbers, such as counting from 1 to 10:
C:\DEVEL\WATCOMC>seq
1
2
3
4
5
6
7
8
9
10
You can also provide your own start, stop, and step values on the command line to generate a different sequence of numbers:
C:\DEVEL\WATCOMC>seq 10 50 5
10
15
20
25
30
35
40
45
50
Programming is fun
Knowing a bit about programming can be a fun way to explore operating systems like FreeDOS. By writing a short program, you can create your own useful tools to solve all kinds of problems, or to make the command line more useful to you.
This example used C to create a useful program. If you prefer Assembly, check out the Flat Assembler (FASM), JWASM, TinyASM, or the Netwide Assembler (NASM). For BASIC programming, try the Bywater BASIC interpreter or the FreeBASIC compiler. FreeDOS also includes compilers and tools for other languages such as Javascript, Lua, Perl, FORTRAN77, and Pascal. Use the FDIMPLES
package manager to install your favorite compiler from the “Development” package group in the FreeDOS distribution.
More from We Love Open Source
- 5 FreeDOS editors I love
- How to navigate the command line
- Explore the five steps of the FreeDOS boot sequence
- A throwback experiment with Linux and Unix
The opinions expressed on this website are those of each author, not of the author's employer or All Things Open/We Love Open Source.