We ❤️ Open Source
A community education resource
How to make a web color palette with Bash tutorial
Use a ‘for’ loop in Bash to loop over a set of values and create a web-safe color palette.
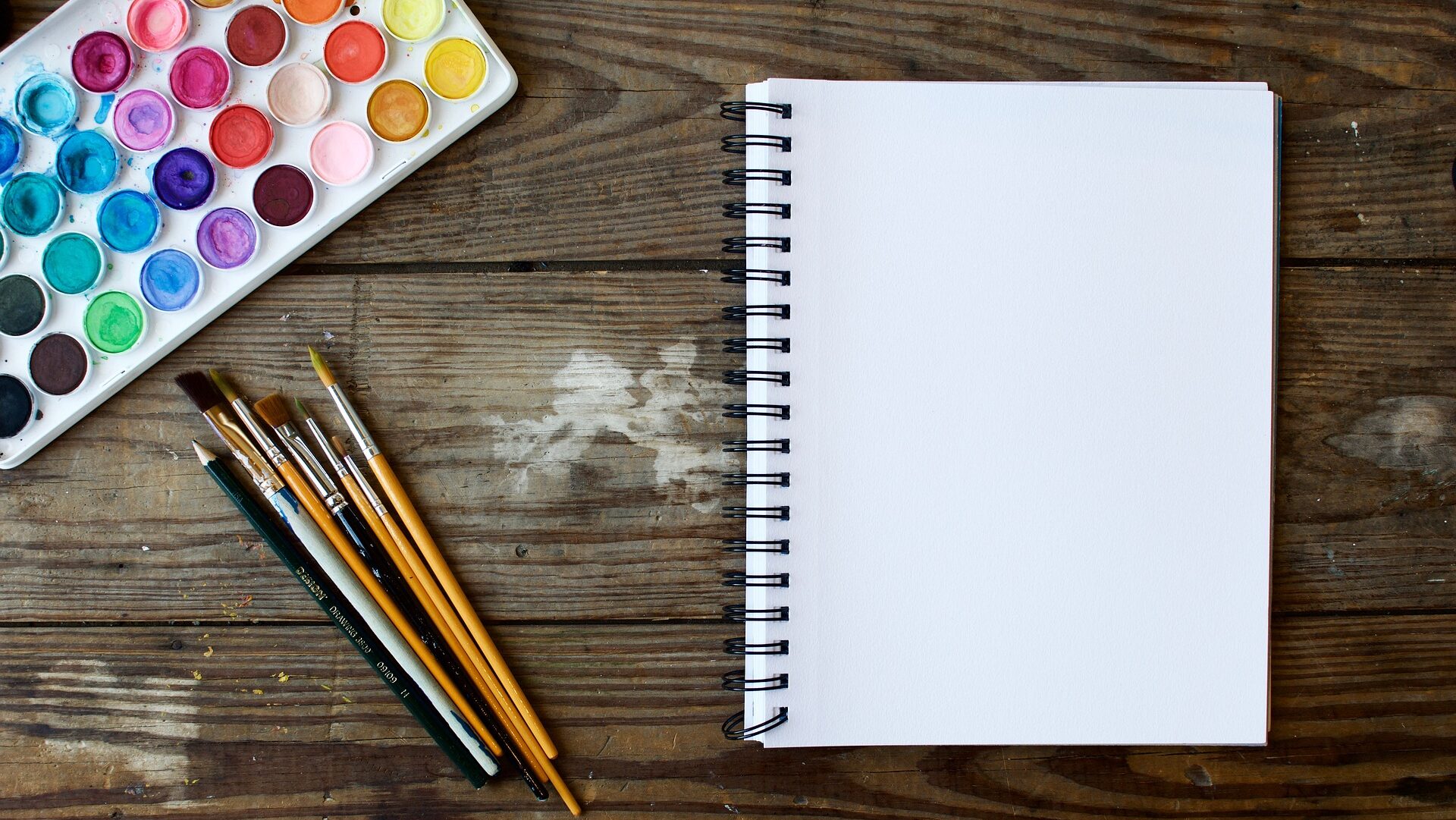
You can select colors in HTML pages using several different methods, including the old standby of “hex” or hexadecimal color values. Specifying a color using hex values requires a combination of numbers that represent the amount of red, green, and blue light. These values are in the range 0 to 255, but in hex numbers that’s 00
to ff
.
When the web was still a new idea, and computers had only a limited set of colors they could display reliably across different systems, many web developers used a set of “web-safe colors” to design new websites. These web-safe colors incremented the hex values by “3” so had numbers like 00
, 33
, .. and so on until cc
and ff
. While modern computers can display many more colors than this limited palette, I sometimes like to refer back to the web-safe colors when I create new web pages. That way, I know my pages will look good anywhere.
You can find copies of the web-safe palette in many places online, but you can just as easily create your own web-safe color sample. And it’s a perfect opportunity to learn how to use the for loop in Bash scripts.
How to use the Bash ‘for’ loop
The basic form of a Bash for loop looks like this:
for variable in set ; do statements ; done
For example, let’s say you wanted to print the numbers 1, 2, and 3. You can do that using a Bash for
loop:
$ for n in 1 2 3; do echo $n; done
1
2
3
Bash uses semicolons to separate commands, and let you write multiple statements on a single line. If you were write this for
loop out in a Bash script file, you might instead write it on multiple lines like this:
#!/bin/bash
for n in 1 2 3
do
echo $n
done
But I like to write out my for
loops so the do
starts “inline” with the for
, and the done
statement starts at the same level as the for
statement:
#!/bin/bash
for n in 1 2 3 ; do
echo $n
done
However you choose to write out your for
statement is a matter of personal preference.
Nested loops
You can also put one loop inside another loop, called “nested” loops. This can help you iterate over two or more variables, to combine tasks that need to be done at the same time. For a simple example, let’s say you wanted to print the combinations of the letters A, B, and C with the numbers 1, 2, and 3. You can do that with two nested for
loops in Bash:
#!/bin/bash
for letter in A B C ; do
for num in 1 2 3 ; do
echo $letter$num
done
done
This Bash script generates the nine combinations of the three letters and three numbers:
$ bash nested.bash
A1
A2
A3
B1
B2
B3
C1
C2
C3
Creating the web color palette with a ‘for’ loop
The web-safe color palette is all possible combinations of red, green, and blue from the hex color #000000
(black) to #ffffff
(white) where each hexadecimal value of red, green, and blue steps up between the values 00
, 33
, 66
, 99
, cc
and ff
. To create the web-safe palette, we need to use three nested for loops, each for red, green, and blue values:
#!/bin/bash
for red in 00 33 66 99 cc ff ; do
for green in 00 33 66 99 cc ff ; do
for blue in 00 33 66 99 cc ff ; do
echo "#$red$green$blue"
done
done
done
Saving this script as colors.bash and running it generates a list of all 216 possible combinations of web-safe colors:
$ bash colors.bash | wc -l
216
$ bash colors.bash | head
#000000
#000033
#000066
#000099
#0000cc
#0000ff
#003300
#003333
#003366
#003399
Making it look pretty
We can use this script to generate an HTML page with each of the web-safe colors, arranged in a “palette.” To do that, let’s first make a small edit to the Bash script, to put each color in a separate <div>
and each hex code inside a <code>
tag. This will make it easier to apply styles to the HTML page in the next step:
#!/bin/bash
for red in 00 33 66 99 cc ff ; do
for green in 00 33 66 99 cc ff ; do
for blue in 00 33 66 99 cc ff ; do
echo "<div style='background-color:#$red$green$blue'>"
echo "<code>#$red$green$blue</code>"
echo "</div>"
done
done
done
This updated Bash script generates an HTML page with “bands” of colors:
$ bash colors.bash > colors.html
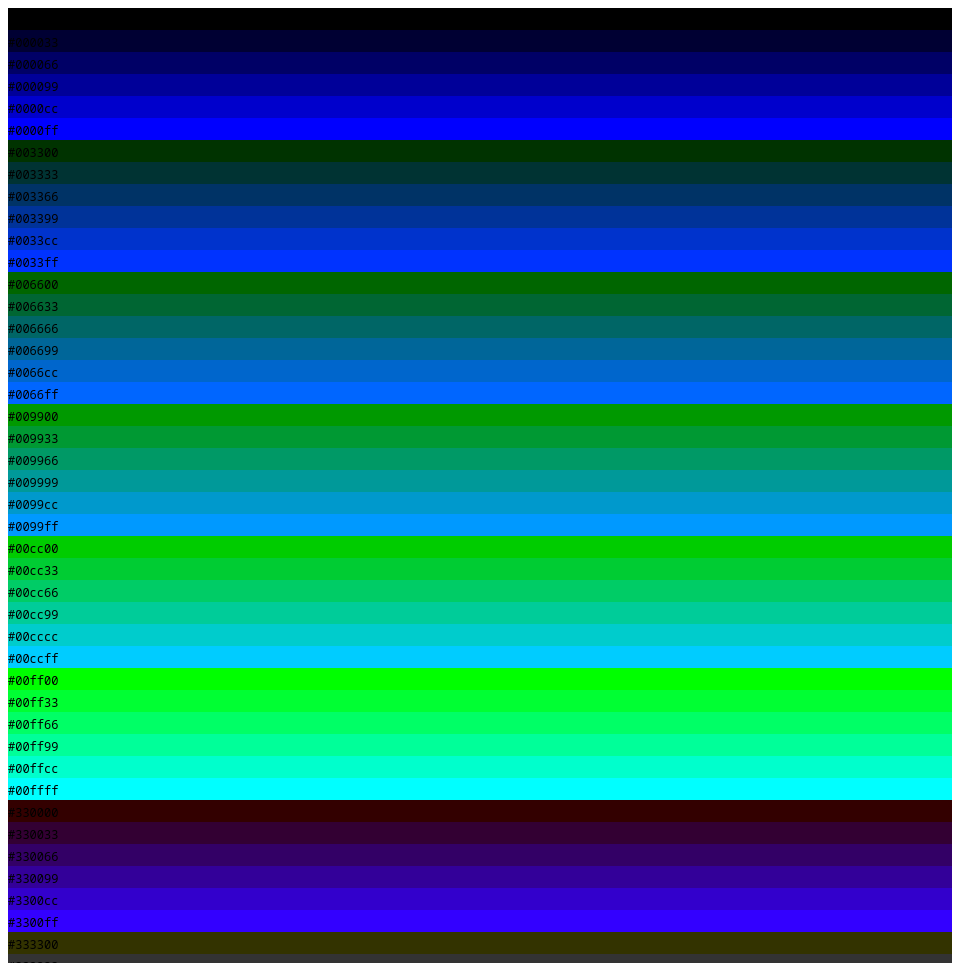
To turn the color bands into boxes, we need to apply a CSS style called “grid” to place the <div>
elements in a grid of six boxes per row. At the same time, we can add “padding” inside each box so the color and hex code are easier to see, and a small “gap” between each box in the grid. An updated Bash script might look like this, with the extra HTML and CSS inserted in the script using a Bash trick called “here” documents, using cat<<EOF
to start the “here” document, ended with EOF
on its own line:
#!/bin/bash
cat<<EOF
<!DOCTYPE html>
<html>
<head>
<title>Web-safe colors</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
@media only screen and (min-width:700px) {
body {
display: grid;
grid-template-columns: repeat(6,1fr);
column-gap: 1em;
row-gap: 1em;
}
div {
padding-bottom: 2em;
}
}
code {
background-color: black;
color: white;
}
</style>
</head>
<body>
EOF
for red in 00 33 66 99 cc ff ; do
for green in 00 33 66 99 cc ff ; do
for blue in 00 33 66 99 cc ff ; do
echo "<div style='background-color:#$red$green$blue'>"
echo "<code>#$red$green$blue</code>"
echo "</div>"
done
done
done
cat<<EOF
</body>
</html>
EOF
The Bash script generates a professional-looking web-safe color palette. This makes a handy reference when you need to refer to the web-safe colors. Whenever you need it, just run the script and save the output to a file, then open the file in your web browser:
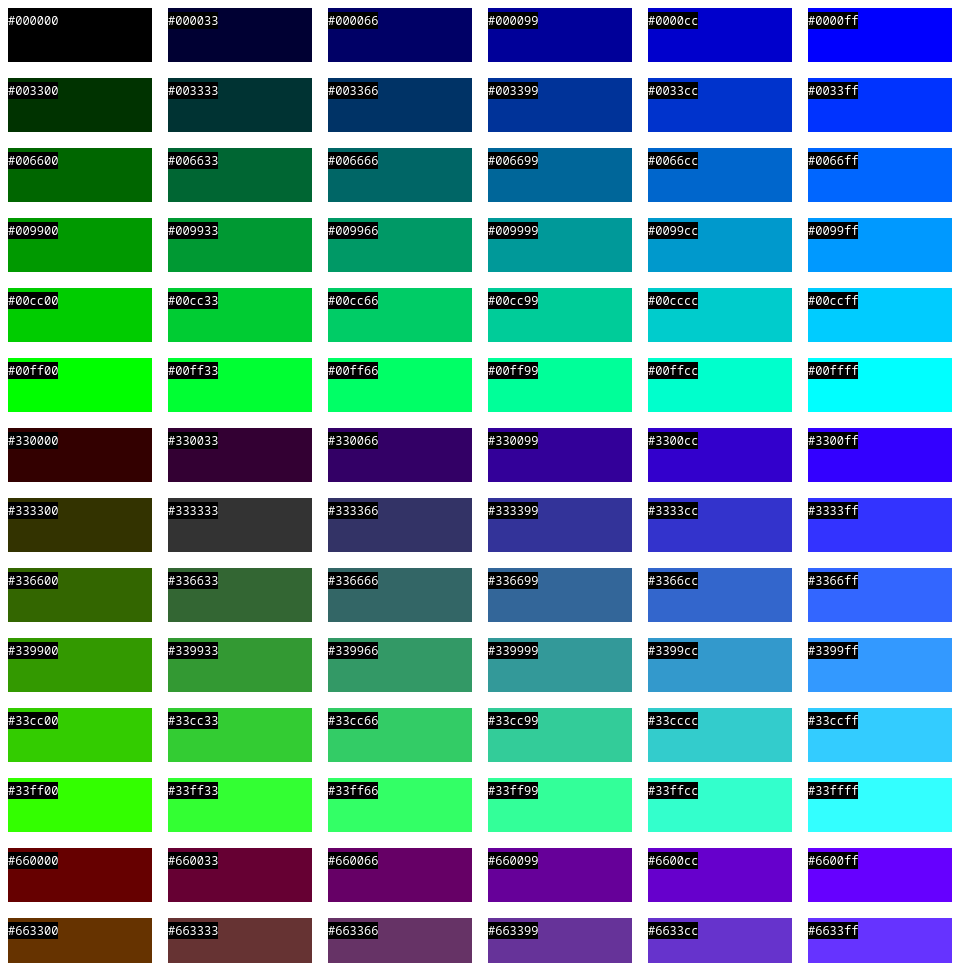
The opinions expressed on this website are those of each author, not of the author's employer or All Things Open/We Love Open Source.